Difficult:Medium
題目
Given the head of a linked list, rotate the list to the right by k places.
翻譯
給定鍊錶的頭部,將鍊錶向右旋轉 k 位。
範例
Example 1
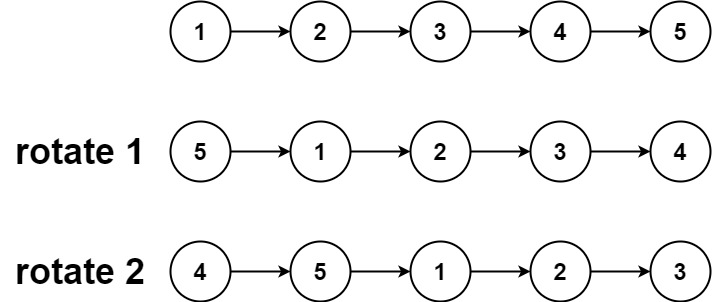
1 2
| Input: head = [1,2,3,4,5], k = 2 Output: [4,5,1,2,3]
|
Example 2
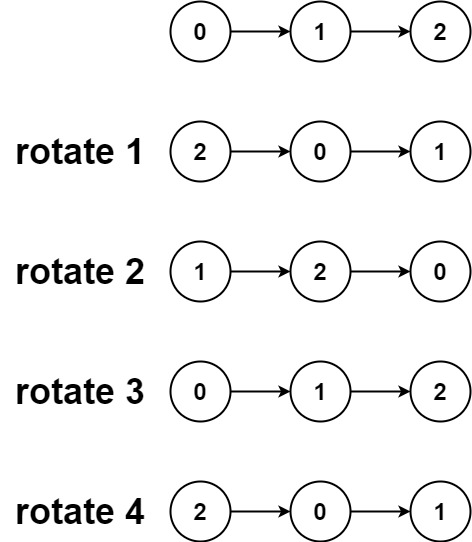
1 2
| Input: head = [0,1,2], k = 4 Output: [2,0,1]
|
解題思路
1.先算出node長度
2.反轉node將最後的依序往前
Solution
Code 1 :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| var rotateRight = function (head, k) { if (head == null || head.next == null || k == 0) { return head; } let headnode = head; let curnode = headnode; let prenode; let length = 1; while (curnode.next != null) { prenode = curnode; curnode = curnode.next; length++; } prenode.next = null; curnode.next = headnode; headnode = curnode; k = (k - 1) % length; for (let i = 0; i < k; i++) { while (curnode.next != null) { prenode = curnode; curnode = curnode.next; } prenode.next = null; curnode.next = headnode; headnode = curnode; } return curnode; };
|